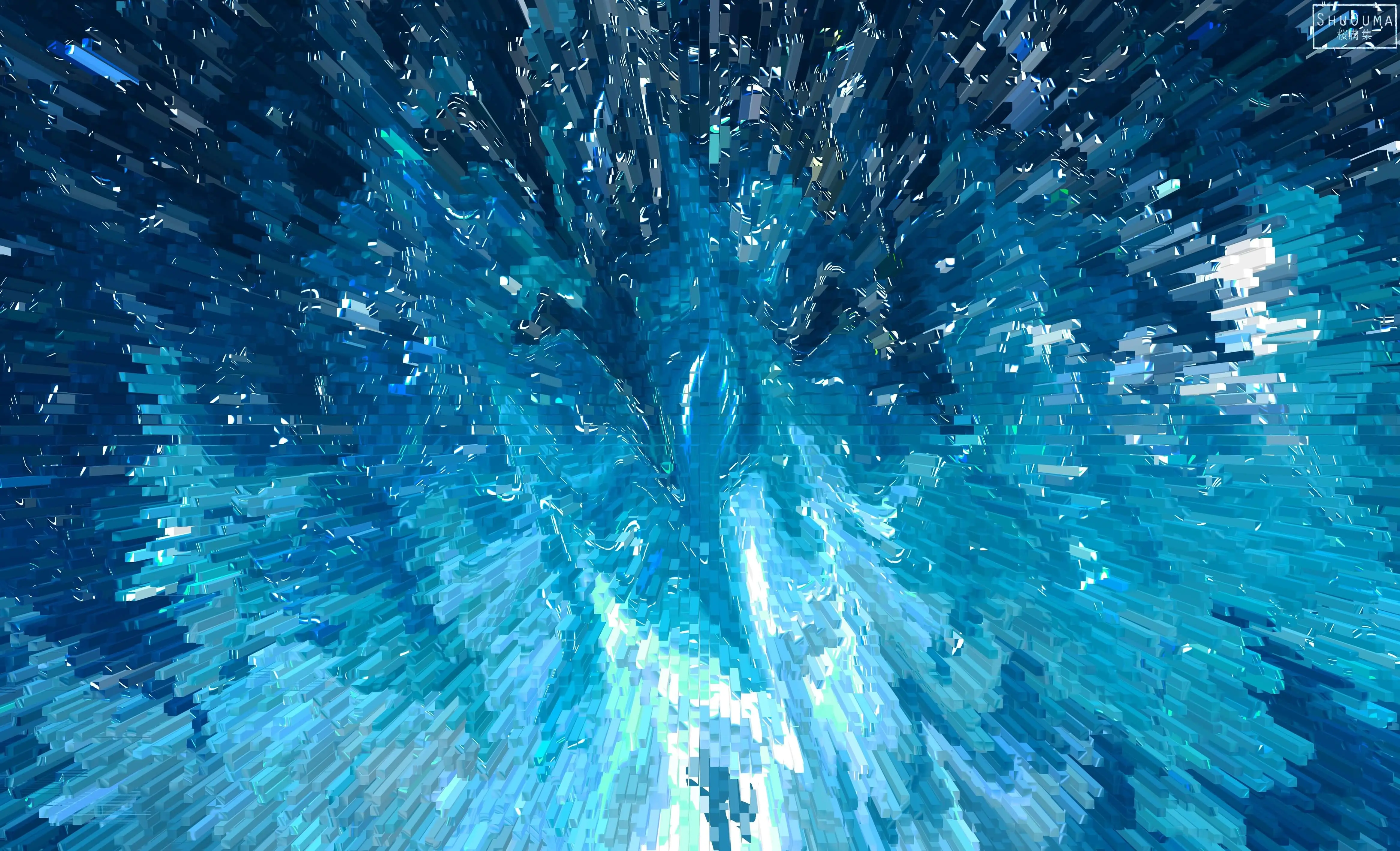
SpringBoot 常用注解
Controller
1、@RestController
@RestController 相当于@Controller+@ResponseBody
@ResponseBody 注解用于将 Controller 的方法返回的对象,通过适当的 HttpMessageConverter 转换为指定格式后,写入到 Response 对象的 body 数据区,通常用来返回 JSON 或者 XML 数据,返回 JSON 数据的情况比较多。
2、@RequestMapping
@RequestMapping 注解是用来映射请求的,即指明 Controller 可以处理哪些 URL 请求,该注解既可以用在类上,也可以用在方法上
3、@PathVariable
用于获取 url 中的参数,比如 user/1 中 1 的值
通过 @PathVariable 可以将 URL 中占位符参数绑定到控制器处理方法的入参中、
URL 中的 {xxx} 占位符可以通过@PathVariable(“xxx“) 绑定到操作方法的入参中。
4、@GetMapping @PostMapping @PutMapping @DeleteMapping
@GetMapping 是 @RequestMapping(method = RequestMethod.GET) 的缩写,只能处理 get 请求
@PostMapping 是 @RequestMapping(method = RequestMethod.POST) 的缩写,只能处理 post 请求
@PutMapping 是 @RequestMapping(method = RequestMethod.PUT) 的缩写,只能处理 put 请求
@DeleteMapping 是 @RequestMapping(method = RequestMethod.DELETE) 的缩写,只能处理 delete 请求
详情见:REST 风格的 URL
范例:
1 | /** |
5、@RequestBody
将客户端请求过来的 json 转成 java 对象
注解@RequestBody 接收的参数是来自 requestBody
中,即请求体。一般用于处理Content-Type: 为 非 Content-Type: application/x-www-form-urlencoded 编码格式的数据,比如:application/json、application/xml
等类型的数据
注意:GET 请求中,如果发出的请求中请求体为空,@RequestBody 并不适用
通过@RequestBody 可以解析 Body 中 json 格式的数据,后端可以通过 @RequestBody + 实体对象来接收
6、@RequestParam
当表单参数和方法形参名字不一致时,做一个名字映射
用来处理 Content-Type: 为 application/x-www-form-urlencoded 编码的内容。(Http 协议中,如果不指定 Content-Type,则默认传递的参数就是 application/x-www-form-urlencoded 类型)
RequestParam 可以接受简单类型的属性,也可以接受对象类型。
实质是将 Request.getParameter() 中的 Key-Value 参数 Map 利用 Spring 的转化机制 ConversionService 配置,转化成参数接收对象或字段。在 Content-Type: application/x-www-form-urlencoded 的请求中,get 方式中 queryString 的值(即暴露在 url 中通过?参数=value),和 post 方式中 body data 的值都会被 Servlet 接受到并转化到 Request.getParameter()参数集中,所以@RequestParam 可以获取的到。
1 |
|
当前请求参数名和形参名一致时,可以省略 @RequestParam
,但是从代码可读性角度来说最好还是写上
比如前端传来的参数:
后端的形参名和前端传来的请求参数名一致,可以省略 @RequestParam