Jedis
1、一个对比
| MySQL | Redis |
---|
连接 | Connection | Jedis |
连接池 | C3P0 等等 | JedisPool |
操作完成 | 关闭连接 | 关闭连接 |
2、Redis 准备
① 理解 Redis 配置文件中 bind 配置项含义
bind 后面跟的 ip 地址是客户端访问 Redis 时使用的 IP 地址。看下面例子:
bind 值 | 访问方式 |
---|
127.0.0.1 | ./redis-cli -h 127.0.0.1 |
192.168.200.100 | ./redis-cli -h 192.168.200.100 |
② 查看 Linux 系统本机 IP
远程客户端访问 Linux 服务器时不能使用 127.0.0.1,要使用网络上的实际 IP。可以用 ifconfig 命令查看。
③ 将 Redis 配置文件中的 bind 配置项设置为本机 IP。
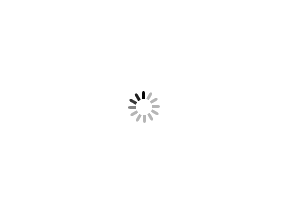
重启 Redis 服务,客户端登录
1
| /usr/local/redis/bin/redis-cli -h 192.168.88.88
|
[root@ShiGuang ~]# /usr/local/redis/bin/redis-cli -h 192.168.88.88
192.168.88.88:6379>
3、新建一个 Maven 工程
配置 pom.xml 文件
1 2 3 4 5 6 7 8 9 10 11 12 13
| <dependencies> <dependency> <groupId>redis.clients</groupId> <artifactId>jedis</artifactId> <version>2.9.0</version> </dependency> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> <scope>test</scope> </dependency> </dependencies>
|
在 test 里面新建一个 java 进行测试
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| package com.atguigu.jedis.test;
import org.junit.Test; import redis.clients.jedis.Jedis; import redis.clients.jedis.JedisPool;
import java.util.List;
public class MyJedisTest {
private String host = "192.168.88.88";
@Test public void testJedis() {
Jedis jedis = new Jedis(host, 6379);
String ping = jedis.ping(); System.out.println("ping = " + ping);
jedis.close();
}
|
使用连接池进行测试
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| @Test public void testJedisPool() {
JedisPool jedisPool = new JedisPool(host, Protocol.DEFAULT_PORT);
Jedis jedis = jedisPool.getResource();
String ping = jedis.ping(); System.out.println("ping = " + ping);
jedis.close(); }
|