新建 SpringBoot 项目: springboot_jpa
配置 pom.xml 文件
1 2 3 4 5 6 7 8 9 10 11
| <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency>
<dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency>
|
配置 application.yml 文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| logging: level: com.atguigu.dao: debug spring: datasource: username: root password: root url: jdbc:mysql://192.168.88.88:3306/springboot?useUnicode=true&characterEncoding=utf8 driver-class-name: com.mysql.cj.jdbc.Driver
redis: port: 6379 host: 192.168.88.88
jpa: database: mysql show-sql: true generate-ddl: true hibernate: ddl-auto: update naming_strategy: org.hibernate.cfg.ImprovedNamingStrategy
server: port: 1888
|
创建实体配置实体
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| package com.atguigu.domain;
import javax.persistence.*;
@Entity @Table(name = "user") public class User {
@Id @GeneratedValue(strategy = GenerationType.IDENTITY) @Column(name = "id") private Long id; @Column(name = "username") private String username; @Column(name = "password") private String password; @Column(name = "name") private String name;
}
|
Controller 类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| package com.atguigu.controller;
import com.atguigu.domain.User; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController @RequestMapping("/user") public class UserController {
@Autowired private UserService userService;
@RequestMapping("/findAll") public List<User> findAll() { return userService.findUsers(); } }
|
Service 类
创建 UserService 接口
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| package com.atguigu.service;
import com.atguigu.domain.User;
import java.util.List;
public interface UserService { List<User> findUsers(); }
|
创建实现类
1 2 3 4 5 6 7 8 9 10 11
| @Service public class UserServiceImpl implements UserService {
@Autowired private UserDao userDao;
@Override public List<User> findUsers() { return userDao.findAll(); } }
|
UserRepository
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| package com.atguigu.dao;
import com.atguigu.domain.User; import org.springframework.data.jpa.repository.JpaRepository;
public interface UserDao extends JpaRepository<User, Integer> {
}
|
测试
浏览器访问:http://localhost:1888/user/findAll
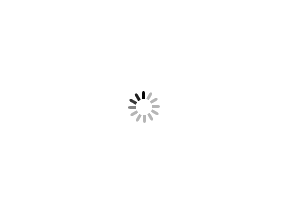
SpringBoot整合Spring Data JPA