一、搭建生产者工程
创建SpringBoot 项目:producer-springboot
1、添加依赖
1 2 3 4 5 6 7 8 9 10 11 12
| <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-amqp</artifactId> </dependency>
<dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> <scope>test</scope> </dependency>
|
2、配置文件
配置application.yml
配置文件
1 2 3 4 5 6 7
| spring: rabbitmq: host: localhost port: 5672 username: guest password: guest virtual-host: /
|
3、创建配置类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| package com.atguigu.config;
import org.springframework.amqp.core.*; import org.springframework.beans.factory.annotation.Qualifier; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration;
@Configuration public class RabbitMQConfig { public static final String EXCHANGE_NAME = "boot_topic_exchange"; public static final String QUEUE_NAME = "boot_queue";
@Bean("bootExchange") public Exchange bootExchange() { return ExchangeBuilder.topicExchange(EXCHANGE_NAME).durable(true).build(); }
@Bean("bootQueue") public Queue bootQueue() { return QueueBuilder.durable(QUEUE_NAME).build(); }
@Bean public Binding bindQueueExchange(@Qualifier("bootQueue") Queue queue, @Qualifier("bootExchange") Exchange exchange) { return BindingBuilder.bind(queue).to(exchange).with("boot.#").noargs(); } }
|
4、发送消息
在 test 目录下创建测试文件 ProducerTest.java
1 2 3 4 5 6 7 8 9 10 11
| @SpringBootTest @RunWith(SpringRunner.class) public class ProducerTest { @Autowired private RabbitTemplate rabbitTemplate;
@Test public void testSend() { rabbitTemplate.convertAndSend(RabbitMQConfig.EXCHANGE_NAME, "boot.hello", "明天是端午节"); } }
|
运行 testSend 测试,查看消息
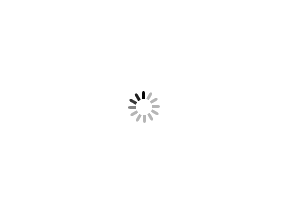
二、搭建消费者工程
创建SpringBoot 项目: consumber-springboot
1、添加依赖
1 2 3 4 5
| <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-amqp</artifactId> </dependency>
|
2、配置文件
配置application.yml
配置文件
1 2 3 4 5 6 7
| spring: rabbitmq: host: localhost port: 5672 username: guest password: guest virtual-host: /
|
3、消息监听器
队列监听器
创建 RabbimtMQListener.java
1 2 3 4 5 6 7 8 9 10 11 12 13
| package com.atguigu.listener;
import org.springframework.amqp.core.Message; import org.springframework.amqp.rabbit.annotation.RabbitListener; import org.springframework.stereotype.Component;
@Component public class RabbimtMQListener { @RabbitListener(queues = "boot_queue") public void listenerQueue(Message message) { System.out.println(new String(message.getBody())); } }
|
4、接收消息
运行 ConsumberSpringbootApplication 主程序
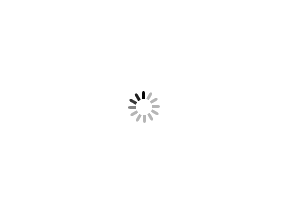