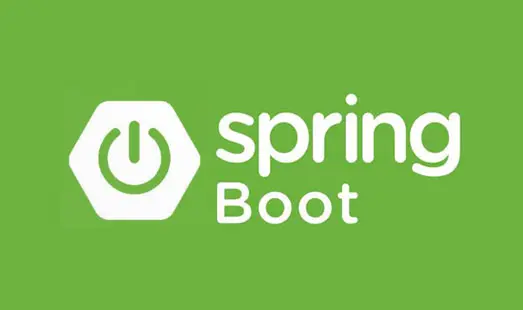
SpringBoot整合定时任务
创建 springboot 项目 springboot_task
pom 文件添加依赖
1 | <!-- 添加 Scheduled 坐标 --> |
主程序添加注解
1 |
|
创建 Controller
1 |
|
修改程序 ,换成 cron
参数
关于cron
表达式:点我查看
1 | // 每隔10秒执行一次 |
运行主程序,查看效果
总结
1、注解
定时任务在配置类上添加@EnableScheduling
开启对定时任务的支持
在相应的任务的类上添加@Component
在相应的方法上添加@Scheduled
声明需要执行的定时任务
2、参数
@Scheduled 注解中有以下几个参数
cron
zone
fixedDelay 和 fixedDelayString
fixedRate 和 fixedRateString
initialDelay 和 initialDelayString
cron 是设置定时执行的表达式,如 0 0/5 * * * ?每隔五分钟执行一次
zone 表示执行时间的时区
fixedDelay 和 fixedDelayString 表示一个固定延迟时间执行,上个任务完成后,延迟多长时间执行
fixedRate 和 fixedRateString 表示一个固定频率执行,上个任务开始后,多长时间后开始执行
initialDelay 和 initialDelayString 表示一个初始延迟时间,第一次被调用前延迟的时间
本文采用 CC BY-NC-SA 4.0 许可协议。转载请注明来自 ShiGuang
评论